Json.NET will call that method during serialization to determine whether or not to serialize the corresponding object member. If your method returns true, the member will be serialized; otherwise, it won't. It's totally up to you how you make the decision whether to return true or false.
I have some data in a C# DataSet object. I can serialize it right now using a Json.net converter like this
However, this uses the property names from data
when printing to the .json file. I would like to change the property names to be something different (say, change 'foo' to 'bar').
In the Json.net documentation, under 'Serializing and Deserializing JSON' → 'Serialization Attributes' it says 'JsonPropertyAttribute... allows the name to be customized'. But there is no example. Does anyone know how to use a JsonPropertyAttribute to change the property name to something else?
(Direct link to documentation)
Json.net's documentation seems to be sparse. If you have a great example I'll try to get it added to the official documentation.Thanks!
Uwe Keim3 Answers
Newtonsoft Json Serialize Name List
You could decorate the property you wish controlling its name with the [JsonProperty]
attribute which allows you to specify a different name:
Documentation: Serialization Attributes
Martin BrownNewtonsoft Json Serialize Name List
If you don't have access to the classes to change the properties, or don't want to always use the same rename property, renaming can also be done by creating a custom resolver.
For example, if you have a class called MyCustomObject
, that has a property called LongPropertyName
, you can use a custom resolver like this…
Then call for serialization and supply the resolver:
And the result will be shortened to {'Short':'prop value'} instead of {'LongPropertyName':'prop value'}
More info on custom resolvers here
Luke GirvinThere is still another way to do it, which is using a particular NamingStrategy, which can be applied to a class or a property by decorating them with [JSonObject]
or [JsonProperty]
.
There are predefined naming strategies like CamelCaseNamingStrategy
, but you can implement your own ones.
The implementation of different naming strategies can be found here: https://github.com/JamesNK/Newtonsoft.Json/tree/master/Src/Newtonsoft.Json/Serialization
JotaBeJotaBeNot the answer you're looking for? Browse other questions tagged c#serializationjson.net or ask your own question.
I have a class that contains an enum
property, and upon serializing the object using JavaScriptSerializer
, my json result contains the integer value of the enumeration rather than its string
'name'. Is there a way to get the enum as a string
in my json without having to create a custom JavaScriptConverter
? Perhaps there's an attribute that I could decorate the enum
definition, or object property, with?
As an example:
Desired json result:
Ideally looking for answer with built-in .NET framework classes, if not possible alternatives (like Json.net) are welcome.
Alexei Levenkov24 Answers
No there is no special attribute you can use. JavaScriptSerializer
serializes enums
to their numeric values and not their string representation. You would need to use custom serialization to serialize the enum
as its name instead of numeric value.
If you can use JSON.Net instead of JavaScriptSerializer
than see answer on this question provided by OmerBakhari: JSON.net covers this use case (via the attribute [JsonConverter(typeof(StringEnumConverter))]
) and many others not handled by the built in .net serializers. Here is a link comparing features and functionalities of the serializers.
I have found that Json.NET provides the exact functionality I'm looking for with a StringEnumConverter
attribute:
More details at available on StringEnumConverter
documentation.
There are other places to configure this converter more globally:
enum itself if you want enum always be serialized/deserialized as string:
In case anyone wants to avoid attribute decoration, you can add the converter to your JsonSerializer (suggested by Bjørn Egil):
and it will work for every enum it sees during that serialization (suggested by Travis).
or JsonConverter (suggested by banana):
Additionally you can control casing and whether numbers are still accepted by using StringEnumConverter(NamingStrategy, Boolean) constructor.
Alexei LevenkovAdd the below to your global.asax for JSON serialization of c# enum as string
C# Json Serialize Namevaluecollection
IggyIggy@Iggy answer sets JSON serialization of c# enum as string only for ASP.NET (Web API and so).
But to make it work also with ad hoc serialization, add following to your start class (like Global.asax Application_Start)
More information on the Json.NET page
Additionally, to have your enum member to serialize/deserialize to/from specific text, use the
System.Runtime.Serialization.EnumMember
attribute, like this:
JuriJuriI wasn't able to change the source model like in the top answer (of @ob.), and I didn't want to register it globally like @Iggy. So I combined https://stackoverflow.com/a/2870420/237091 and @Iggy's https://stackoverflow.com/a/18152942/237091 to allow setting up the string enum converter on during the SerializeObject command itself:
The combination of Omer Bokhari and uri 's answers is alsways my solution since the values that I want to provide is usually different from what I have in my enum specially that I would like to be able to change my enums if I need to.
So if anyone is interested, it is something like this:
Ashkan SirousAshkan SirousThis is easily done by adding a ScriptIgnore
attribute to the Gender
property, causing it to not be serialised, and adding a GenderString
property which does get serialised:
This version of Stephen's answer doesn't change the name in the JSON:
ASP.NET Core way:
Here is a simple solution that serializes a server-side C# enum to JSON and uses the result to populate a client-side <select>
element. This works for both simple enums and bitflag enums.
I have included the end-to-end solution because I think most people wanting to serialize a C# enum to JSON will also probably be using it to fill a <select>
drop-down.
Here goes:
Example Enum
A complex enum that uses bitwise ORs to generate a permissions system. So you can't rely on the simple index [0,1,2..] for the integer value of the enum.
Server Side - C#
The code above uses the NancyFX framework to handle the Get request. It uses Nancy's Response.AsJson()
helper method - but don't worry, you can use any standard JSON formatter as the enum has already been projected into a simple anonymous type ready for serialization.
Newtonsoft Json Serialize Enum Name
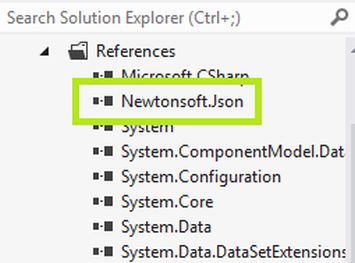
Generated JSON
Client Side - CoffeeScript
HTML Before
HTML After
biofractalbiofractalYou can also add a converter to your JsonSerializer
if you don't want to use JsonConverter
attribute:
It will work for every enum
it sees during that serialization.
For ASP.Net core Just add the following to your Startup Class:
Yahya HusseinYahya HusseinYou can create JsonSerializerSettings with the call to JsonConverter.SerializeObject as below:
Yang ZhangYang ZhangNoticed that there is no answer for serialization when there is a Description attribute.
Here is my implementation that supports the Description attribute.
Enum:
Usage:
This is an old question but I thought I'd contribute just in case. In my projects I use separate models for any Json requests. A model would typically have same name as domain object with 'Json' prefix. Models are mapped using AutoMapper. By having the json model declare a string property that is an enum on domain class, AutoMapper will resolve to it's string presentation.
In case you are wondering, I need separate models for Json serialized classes because inbuilt serializer comes up with circular references otherwise.
Hope this helps someone.
Ales Potocnik HahoninaAles Potocnik HahoninaJust in case anybody finds the above insufficient, I ended up settling with this overload:
You can actually use a JavaScriptConverter to accomplish this with the built-in JavaScriptSerializer. By converting your enum to a Uri you can encode it as a string.
I've described how to do this for dates but it can be used for enums as well. Custom DateTime JSON Format for .NET JavaScriptSerializer.
Sebastian MarkbågeSebastian MarkbågeNot sure if this is still relevant but I had to write straight to a json file and I came up with the following piecing several stackoverflow answers together
It assures all my json keys are lowercase starting according to json 'rules'. Formats it cleanly indented and ignores nulls in the output. Aslo by adding a StringEnumConverter it prints enums with their string value.
Newtonsoft Json Serialize Property Name
Personally I find this the cleanest I could come up with, without having to dirty the model with annotations.
usage:
I have put together all of the pieces of this solution using the Newtonsoft.Json
library. It fixes the enum issue and also makes the error handling much better, and it works in IIS hosted services. It's quite a lot of code, so you can find it on GitHub here: https://github.com/jongrant/wcfjsonserializer/blob/master/NewtonsoftJsonFormatter.cs
You have to add some entries to your Web.config
to get it to work, you can see an example file here:https://github.com/jongrant/wcfjsonserializer/blob/master/Web.config
A slightly more future-proof option
Facing the same question, we determined that we needed a custom version of StringEnumConverter
to make sure that our enum values could expand over time without breaking catastrophically on the deserializing side (see background below). Using the SafeEnumConverter
below allows deserialization to finish even if the payload contains a value for the enum that does not have a named definition, closer to how int-to-enum conversion would work.
Usage:
or
Source:
Background
When we looked at using the StringEnumConverter
, the problem we had is that we also needed passivity for cases when a new enum value was added, but not every client was immediately aware of the new value. In these cases, the StringEnumConverter
packaged with Newtonsoft JSON throws a JsonSerializationException
similar to 'Error converting value SomeString to type EnumType' and then the whole deserialization process fails. This was a deal breaker for us, because even if the client planned on ignoring/discarding the property value that it didn't understand, it still needed to be capable of deserializing the rest of the payload!